Minimizing and Optimizing JavaScript for Better Performance
In today's web-driven world, JavaScript plays a crucial role in creating interactive and dynamic user experiences. However, poorly optimized JavaScript can significantly impact your website's performance, leading to slower load times and frustrated users. Let's explore some essential techniques for minimizing and optimizing JavaScript to boost your website's performance.
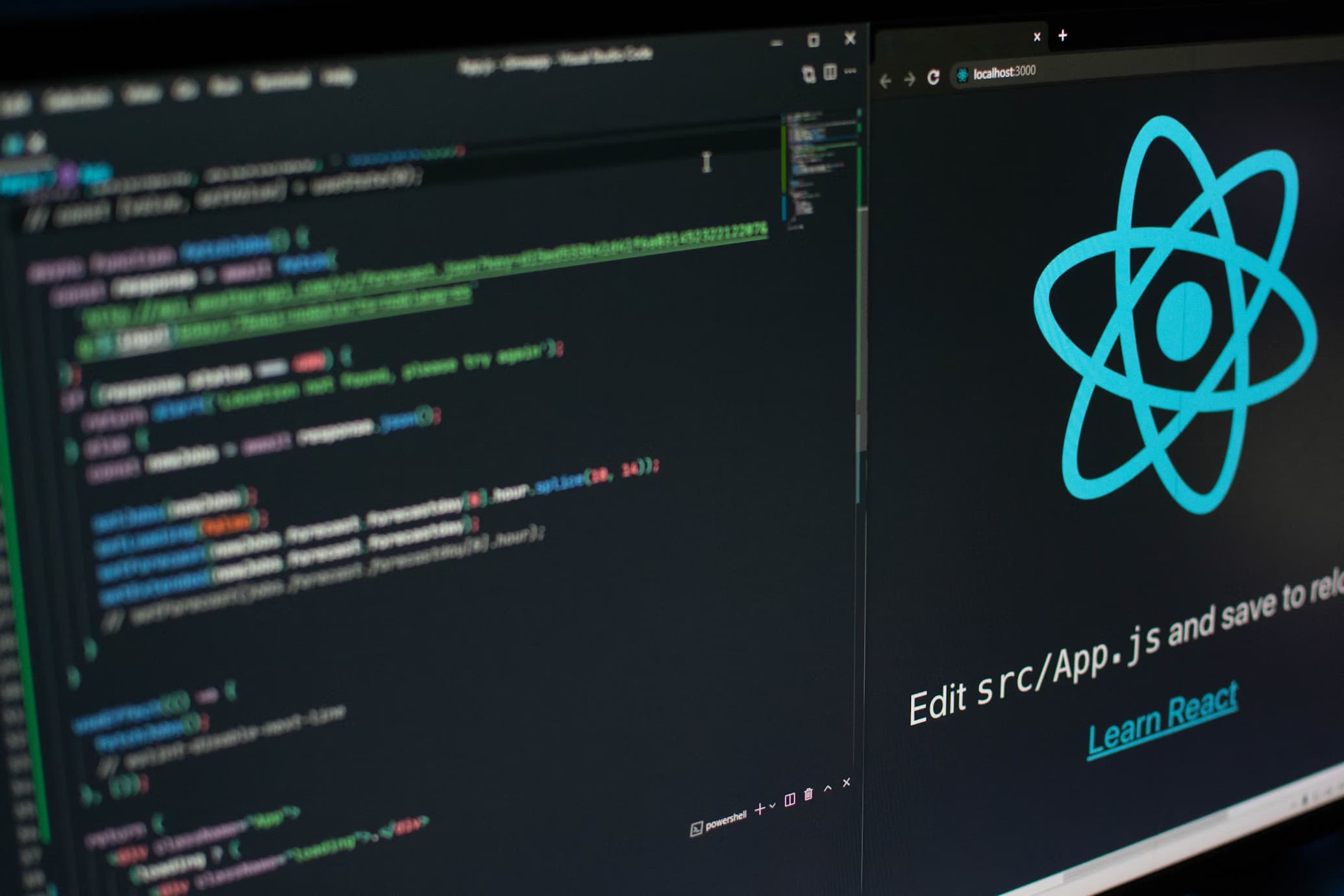
Image source: https://unsplash.com/photos/xkBaqlcqeb4
The Importance of JavaScript Optimization
Before diving into specific techniques, it's crucial to understand why JavaScript optimization matters. According to Google's Web Vitals, JavaScript can significantly impact key performance metrics such as Largest Contentful Paint (LCP) and First Input Delay (FID). Optimizing your JavaScript not only improves these metrics but also enhances overall user experience and can positively impact your search engine rankings.
Code Splitting: Load What You Need, When You Need It
Code splitting is a powerful technique that involves breaking your JavaScript bundle into smaller chunks and loading them on demand. This approach can significantly reduce initial load times, especially for large applications.
Implementing Code Splitting
- Dynamic Imports: Use dynamic
import()
statements to load modules only when they're needed.
button.addEventListener('click', async () => {
const module = await import('./heavy-module.js')
module.doSomething()
})
-
Route-Based Splitting: In single-page applications, split your code based on routes to load only the necessary JavaScript for each page.
-
Component-Based Splitting: For React applications, use
React.lazy()
andSuspense
to dynamically import components:
const HeavyComponent = React.lazy(() => import('./HeavyComponent'))
function MyComponent() {
return (
<React.Suspense fallback={<div>Loading...</div>}>
<HeavyComponent />
</React.Suspense>
)
}
Learn more about code splitting in React's official documentation.
Minification: Reducing File Size
Minification is the process of removing unnecessary characters from your code without changing its functionality. This includes removing whitespace, comments, and shortening variable names.
Tools for Minification
-
Terser: A popular JavaScript parser and mangler/compressor toolkit for ES6+. Learn more about Terser.
-
UglifyJS: Another widely used JavaScript parser, minifier, compressor, and beautifier toolkit. Explore UglifyJS.
-
Webpack: If you're using Webpack, you can use plugins like
TerserPlugin
for minification. Read Webpack's documentation.
Additional Optimization Techniques
-
Tree Shaking: Eliminate dead code by only including the parts of your code that are actually used. This is particularly useful when working with modern JavaScript modules.
-
Compression: Use Gzip or Brotli compression on your server to reduce the size of transferred files further.
-
Caching: Implement proper caching strategies to reduce the frequency of JavaScript downloads for returning visitors.
-
Async and Defer: Use
async
anddefer
attributes on script tags to prevent render-blocking JavaScript.
<script src="non-critical.js" async></script>
<script src="deferred.js" defer></script>
- Optimize Third-Party Scripts: Be cautious with third-party scripts and consider lazy-loading them or using resource hints for critical resources.
Measuring the Impact
To ensure your optimization efforts are paying off, it's essential to measure your website's performance before and after implementing these techniques. Use tools like Google PageSpeed Insights or WebPageTest to get detailed performance metrics and identify areas for further improvement.
Conclusion
Minimizing and optimizing JavaScript is an ongoing process that requires attention to detail and a commitment to performance. By implementing techniques like code splitting and minification, and staying up-to-date with the latest optimization strategies, you can significantly enhance your website's performance, leading to improved user experience and potentially better search engine rankings.
Remember, every millisecond counts in the world of web performance. Start optimizing your JavaScript today and watch your website soar to new heights of speed and efficiency!
Learn more about web performance optimization
By implementing these JavaScript optimization techniques, you'll be well on your way to creating faster, more efficient websites that delight users and stand out in today's competitive digital landscape. Happy optimizing!